Sending "float" data - SUART
- Tasnemul Hasan Nehal
- Nov 17, 2020
- 2 min read
Updated: Nov 19, 2020
Objective: Here, we will learn the basics of how we can establish a SUART serial communication between 2 Arduinos and then send a "float" type data( ex: 55.61 ).
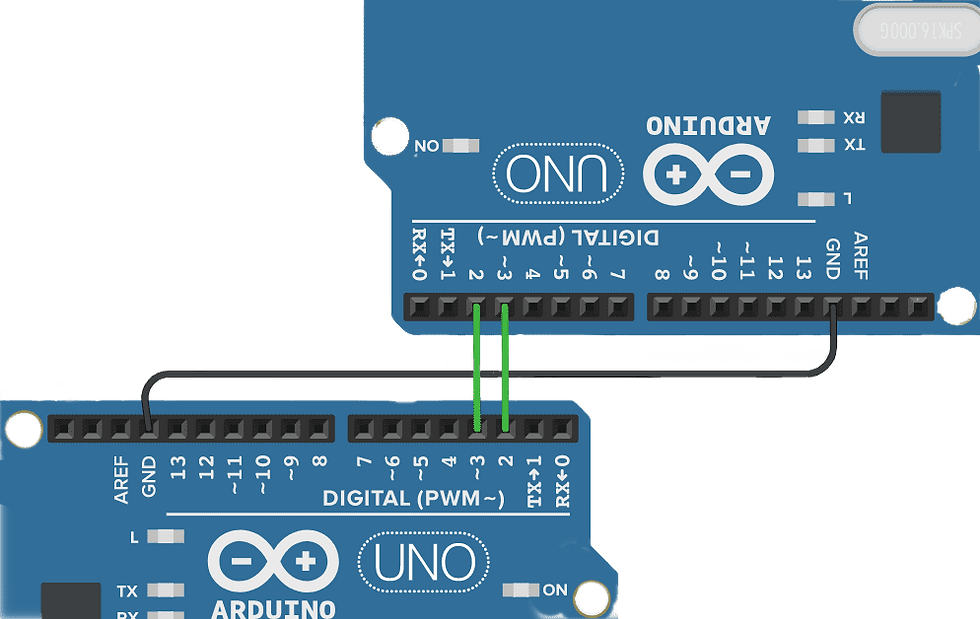
Hardware:
Here for both Arduinos Dpin-2 is working as Receiver and Dpin-3 is working as Transmitter.
Full Code:
ArduinoA (Sender)
#include <SoftwareSerial.h>
SoftwareSerial SUART(2, 3); //Rx(Dpin 2), Tx(Dpin 3)
void intToBytes(int x); //converts integer to bytes
byte b[2]; //converted bytes stored here
void setup()
{
Serial.begin(9600); //Serial Monitor & MCU Communication starts
SUART.begin(9600); //Arduino Uno & Nano Communication starts
}
void loop()
{
float f= 55.61; //we will send this float data
int x= f*100; //converting float to integer
intToBytes(x); //calling user defined function
SUART.write(b[0]); //sending converted bytes data to other device
SUART.write(b[1]);
delay(500);
}
void intToBytes(int x)
{
b[0]= (x >>8); //bitwise "shift" operation
b[1]= x & 0xFF; //bitwise "and" operation
}
ArduinoB (Receiver)
#include <SoftwareSerial.h>
SoftwareSerial mySerial(2, 3); //Rx(Dpin 2), Tx(Dpin 3)
void bytesToInt(byte b0, byte b1); //converts bytes to integer
int x; //converted integer stored here
void setup()
{
Serial.begin(9600); //Serial Monitor & MCU Communication starts
mySerial.begin(9600); //Arduino Uno & Nano Communication starts
}
void loop()
{
while( mySerial.available() > 0 )
{
byte b0 = mySerial.read();
byte b1 = mySerial.read();
bytesToInt(b0, b1); //calling user defined function
float f = (float) x/100;
Serial.println(f);
}
delay(500); //change delay to see differences
}
void bytesToInt(byte b0, byte b1)
{
x = (b0 << 8) | b1; //bitwise operation
}
Code Explanation:
At Sender-
SoftwareSerial SUART(2, 3);
any name can replace "SUART", It works like a constant variable we call it when we need to work with UART Communication. here we used- Rx(Dpin 2), Tx(Dpin 3).
void intToBytes(int x)
Through SUART, we can not directly send the "integer/float" type data. All Serial Communication is only possible with "byte" type data(ex: 0x12). So we first convert the float to integer then integer to bytes. We created this user-defined function which will take an integer input then will split it into 2 data bytes. We use the Bitwise (>>, &) operator to make this happen.
At Receiver-
SoftwareSerial mySerial(2, 3);
same, "mySerial" is just a name, any other name can be used.
void bytesToInt(byte b0, byte b1);
This user-defined function will do reverse engineering. Means this function will take 2 bytes type data as input and convert those to one integer. So at the receiver monitor, we will see the same float data that was sent from other Arduino.
while( mySerial.available() > 0 )
Till there are data coming to the receiver, this loop will run
byte bo = mySerial.read();
byte b1 = mySerial.read();
1st incoming byte data will be read and stored at "b0" and 2nd data at "b1".
Result:
At receiver serial monitor
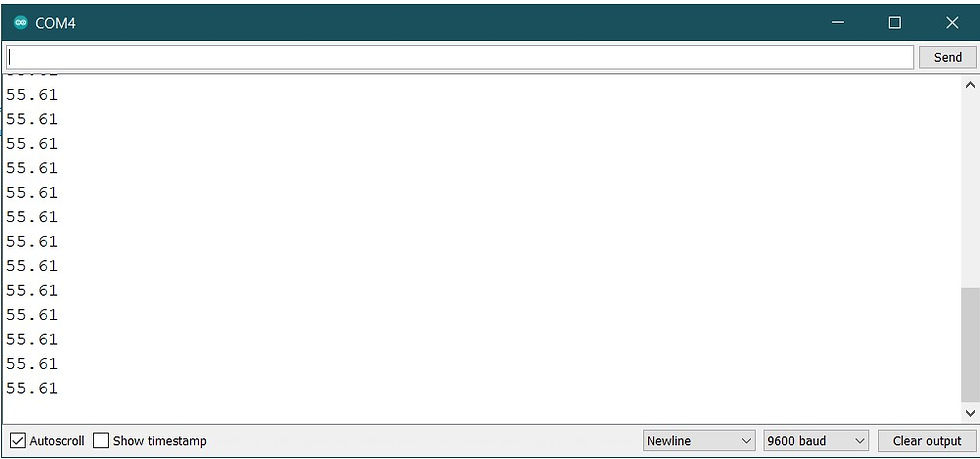
See More Examples:
Comments