Sending "1byte" data - SUART
- Tasnemul Hasan Nehal
- Nov 17, 2020
- 1 min read
Updated: Nov 19, 2020
Objective: Here, we will learn the basics of how we can establish a SUART serial communication between 2 Arduinos and then send 1byte data(ex: 0x5B).
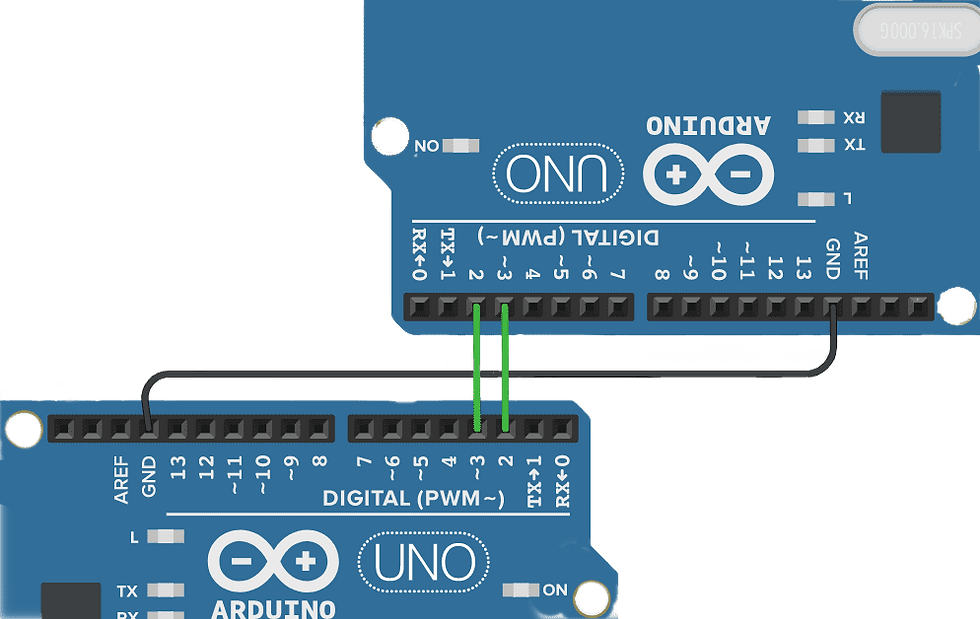
Hardware:
Here for both Arduinos Dpin-2 is working as Receiver and Dpin-3 is working as Transmitter.
Full Code:
ArduinoA (Sender)
#include <SoftwareSerial.h>
SoftwareSerial SUART(2, 3);
void setup()
{
Serial.begin(9600); //Serial Monitor & MCU Communication starts
SUART.begin(9600); //ArduinoA & ArduinoB Communication starts
}
void loop()
{
SUART.write(0x5B); //sending 1byte data(0x56) to other device
delay(10);
}
ArduinoB (Receiver)
#include <SoftwareSerial.h>
SoftwareSerial mySerial(2, 3);
void setup()
{
Serial.begin(9600); //Serial Monitor & MCU Communication starts
mySerial.begin(9600); //Arduino Uno & Nano Communication starts
}
void loop()
{
if( mySerial.available() )
{
byte x = mySerial.read();
//Serial.println(x); //shows decimal of 0x5B = 91
Serial.println(x, HEX); //shows 5B, the actual form
}
delay(10);
}
Code Explanation:
At Sender-
SoftwareSerial SUART(2, 3);
any name can replace "SUART", It works like a constant variable we call it when we need to work with UART Communication. Here we used- Rx(Dpin 2), Tx(Dpin 3).
At Receiverr-
SoftwareSerial mySerial(2, 3);
same, "mySerial" is just a name, any other name can be used.
if( mySerial.available() )
Checks if any serial data is received.
byte x = mySerial.read();
If there is any data, then it will read the data and store it to the x variable. As we are expecting only 1byte data, so x is declared a single-byte type data.
Result:
Serial.println(x); Serial.println(x, HEX);
(decimal) (HexaDecimal)
See More Examples:
Comentarios