Sending "String" data - SUART
- Tasnemul Hasan Nehal
- Nov 17, 2020
- 2 min read
Updated: Nov 19, 2020
Objective: Here, we will learn the basics of how we can establish a SUART serial communication between 2 Arduinos and then send a "String" type data(ex: "Hello There").
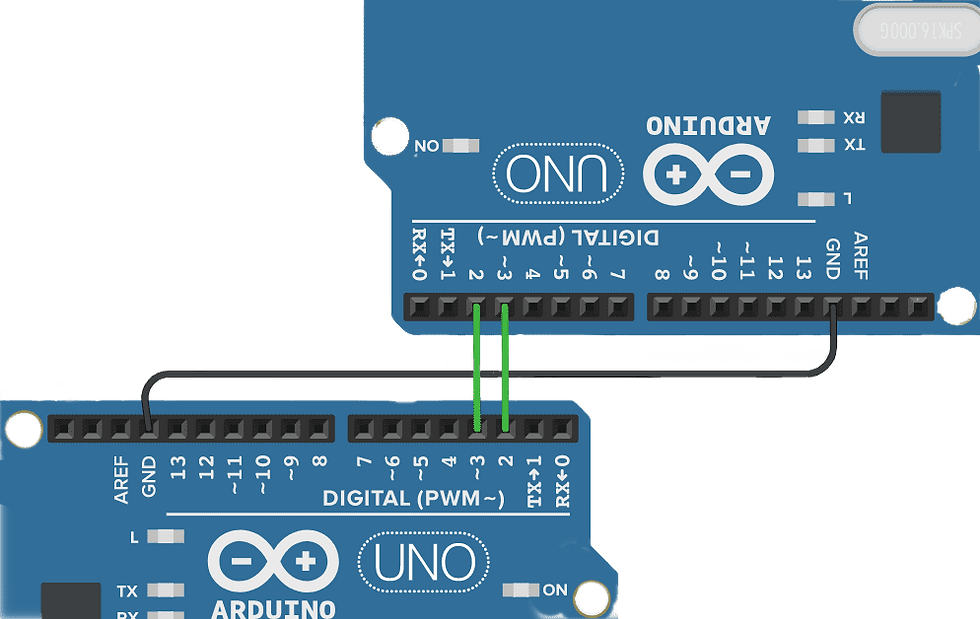
Hardware:
Here for both Arduinos Dpin-2 is working as Receiver and Dpin-3 is working as Transmitter.
Full Code:
ArduinoA (Sender)
#include <SoftwareSerial.h>
SoftwareSerial SUART(2, 3); //Rx(Dpin 2), Tx(Dpin 3)
void setup()
{
Serial.begin(9600); //Serial Monitor & MCU Communication starts
SUART.begin(9600); //Arduino Uno & Nano Communication starts
}
void loop()
{
char x[]= "Hello there"; //the string we want to sent
SUART.write(x, sizeof(x)); //data is sending
SUART.write('\n'); //sending a confirmation 'character'
delay(10);
}
ArduinoB (Receiver)
#include <SoftwareSerial.h>
SoftwareSerial SUART(2, 3); //Rx(Dpin 2), Tx(Dpin 3)
char x[20]; //stores incoming string
void setup()
{
Serial.begin(9600); //Serial Monitor & MCU Communication starts
SUART.begin(9600); //Arduino Uno & Nano Communication starts
}
void loop()
{
while( SUART.available() > 0 )
{
byte m = SUART.readBytesUntil('\n', x,20);
x[m] = '\0'; //m is the size of the string
Serial.println(x); //here x is string
}
}
Code Explanation:
At Sender-
SoftwareSerial SUART(2, 3);
any name can replace "SUART", It works like a constant variable we call it when we need to work with UART Communication. Here we used- Rx(Dpin 2), Tx(Dpin 3).
SUART.write(x, sizeof(x));
Normally we sent only "byte" type data using .write( ) function. But it is also possible to directly send an array of characters (String) by this command. Unlike integer, the size of a 'character' is 1byte, thus it's possible to directly send a character. Since we are sending multiple 'characters' (array) one by one at the same time we need to mention the total data size so that the receiver knows how many memory locations it should reserve for incoming bytes.
SUART.write('\n');
We send this character to confirm the receiver that our main data is sent. When the receiver finds this character ('\n'), it will stop taking any more data. '\n' will be received but won't be stored in the receiver device.
At Receiver-
SoftwareSerial mySerial(2, 3);
same, "mySerial" is just a name, any other name can be used.
char x[20];
Let's say we don't know how many characters will come from the sender, so we assume a maximum of 20 characters can come. No character will be saved after that.
while( SUART.available() > 0 )
We know that data is coming as an array of characters (string) one by one. So we need to keep storing the data until all the characters come. That's why we used a "While( ) " loop instead of an "if ( )" function.
byte m = SUART.readBytesUntil('\n', x,20);
This command will read incoming "byte" data until it finds '\n' character. And all the incoming data will be stored in x variable which can take a maximum of 20 characters. Here the "m" gives us the number of total bytes received. If we send "Hello there", at the receiver m=11.
x[m] = '\0';
After receiving data, x[] will remain a character array but the sender sent a string. So we insert a '\0' (null) character at the end of the x[] to make it a string. For the example of "Hello there". x[0] to x[10] = main data, and x[11] = '\0'
Result:
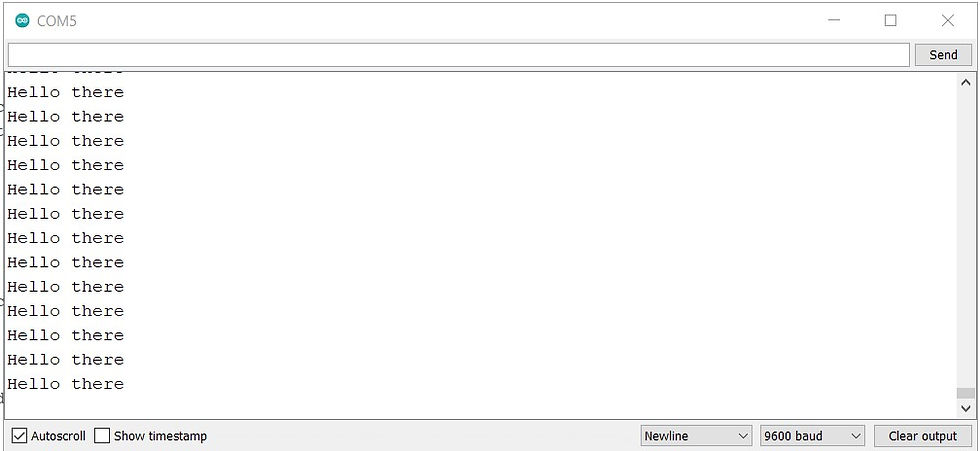
See More Examples:
Comments