Searching specific Slave - I2C bus
- Tasnemul Hasan Nehal
- Nov 17, 2020
- 2 min read
Objective:
Here we will learn how to establish a I2C bus serial communication between 2 Arduinos. One will be Master and another will be Slave. Then we run a search operation to find a specific Slave Arduino.
Theory:
Before we go for coding, we must know the basics of I2C bus and how it works. I highly recommend you to go through Scott Campbell's article on I2C bus to understand it better.
Hardware Setup:
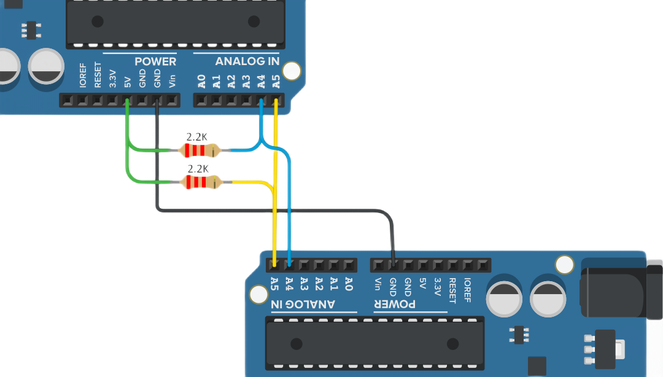
Full Code:
ArduinoA (Master)
#include<Wire.h>
/*
we will search for slaves from no 0x08 to 0x7E (1 increment)
if any slave with any of these address found on the bus, we will print the adress on Master's sserial monitor
*/
byte start_address = 0x08;
byte end_address = 0x7E;
void setup()
{
Wire.begin(); //only for master to create communication
Serial.begin(9600); //MCU & Serial Monitor communication starts
while (start_address <= end_address) //loop from 0x08 to 0x7E
{
byte n = Wire.requestFrom(start_address, 1);
if ( n >0 )
{
Serial.print("Salve found at address : 0x");
Serial.println(start_address, HEX);
}
start_address++;
}
if(start_address > end_address)
{
Serial.print("Done Seraching");
}
}
void loop()
{
;
}
ArduinoB (Slave)
#include<Wire.h>
void setup()
{
Wire.begin(0x4F); //slave address to call by Master
Serial.begin(9600); //MCU & Serial Monitor communication starts
Wire.onRequest(sendData);
}
void loop()
{
;
}
void sendData()
{
;
}
Code Explanation:
At Master
byte n = Wire.requestFrom(start_address, 1);
requesting a particular slave to send 1byte data
byte n = Wire.requestFrom(0x4F, 1);
Requesting Slave no-0x52 send 1byte data. If the slave 0x52 is online on the bus, then n=1, not necessary any data to come. This value "1" is used to allocate memory space for incoming data.
byte n = Wire.requestFrom(0x4F, 5);
Here n=5. Which means the Master allocated 5 bytes of data where incoming data will be stored. Now if 2/3 bytes data comes then the rest of the memory location will be auto-filled with 0xFF (in decimal = 255)
Serial.println(start_address, HEX);
printing slave address that was found through search loop
if(start_address > end_address)
If all the slave from 0x08 to 0x7E is done checking
At Slave
Wire.onRequest(sendData);
When the Master asks to send any data by terminating "Wire.requestFrom(0x4F, 1)" in the Master side code, the "sendData" function will run automatically to send the requested data. This function works only for Slaves. Masters dont have this type of sending function. "sendData" is a regular function name, it can be replaced by any name that follows the Variable naming rules.
Result:
Master's Serial Monitor
Remember we write our code on "void setup( )", that's why the code runs only once.
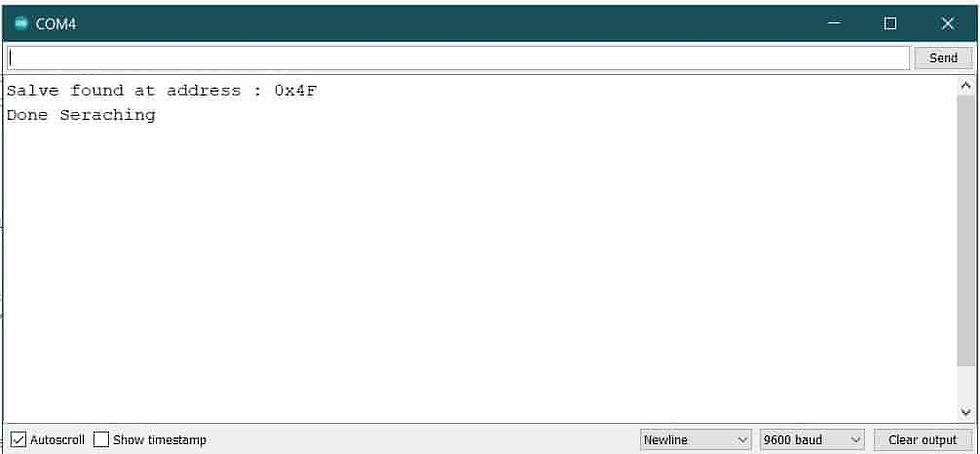
See More Examples:
Commentaires